When faced with the challenge of designing circuits that must make logical decisions, digital circuits are the go-to choice for engineers and hobbyists alike. But why? At the heart of their preference lies the unparalleled ability of digital circuits to precisely and consistently handle vast amounts of information. This feat is achieved through number codes, specifically, the binary and binary-coded decimal (BCD) systems.
Binary numbers, made up of just 0s and 1s, serve as the fundamental language of computers. On the other hand, BCD numbers are a particular type of binary number where each digit of a decimal number is represented separately using its 4-bit binary code.
BCD’s unique setup is instrumental in electronic devices that display numerical data, such as digital clocks or calculators. The reason? BCD's structure aligns well with how display hardware works.
In this article, we'll take a hands-on approach to converting numbers from binary to BCD on the Arduino platform.
Binary Numbers: A Simple Yet Powerful System
There are only two voltage states in digital circuits: high or low, unlike the continuously varying levels in analog circuits. These states signify meanings within a circuit, such as a switch’s on or off state.
Digital circuits represent information using the binary number system, or base-2. This system uses only two symbols: 0 and 1. A binary number is composed of bits. The leftmost bit is the Most Significant Bit (MSB), while the rightmost bit is the Least Significant Bit (LSB). Each bit is represented by powers of two, with the LSB representing 20, and the bit just left to the LSB representing 21, then 22, and so on.
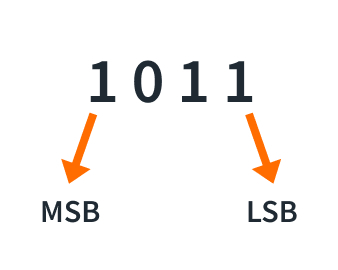
Here's a simple breakdown of the powers of 2 we just talked about:
- 23 : 8
- 22 : 4
- 21 : 2
- 20 : 1
So the binary number (1011)2 translates to:
(1011)2 = 1 x 23 + 0 x 22 + 1 x 21 + 1 x 20 = 8+0+2+1=11 in decimal (base-10).
Binary in Programming and Data Representation
In our world of embedded system design, binary is the foundational language. It's what we use to express all kinds of information - from numeric values and strings of text to complex data like images and audio signals. Regardless of its nature, every byte of data we work with is ultimately boiled down to binary because that's how our microcontrollers, microprocessors, and memory chips deal with and process data.
Advantages of Binary
- Simplicity: Binary represents information in the most simplified form - 'on' (1) or 'off' (0), mirroring the basic architecture of digital computing hardware, which consists of millions or billions of switches that can either allow current to pass (on) or not (off).
- Error Resistance: Binary's two-state system is less prone to errors than multi-state systems.
Converting Between Decimal and Binary
Decimal to Binary
Converting a decimal number to binary is a simple division process. Here's how you can do it:
- Choose a Decimal Number
- Divide by 2
- Write Down the Remainder (either 0 or 1).
- Update the number to be the quotient from the division in step 2.
- Repeat steps 2-4 until the number is 0.
- Write down the remainder in reverse order.
Example:
- 43÷2 = 21 remainder 1
- 21÷2 = 10 remainder 1
- 10÷2 = 5 remainder 0
- 5÷2 = 2 remainder 1
- 2÷2= 1 remainder 0
- 1÷2= 0 remainder 1
So the binary representation of (43)10 is (101011)2
Binary to Decimal
Converting a binary number to a decimal is a straightforward process involving multiplying by powers of 2.
Here's the step-by-step process:
- Choose a Binary Number
- Starting from the right, identify the position of each digit. The rightmost digit (LSB) is position 0; the next is 1, and so on.
- For each position, multiply the binary digit by 2 raised to the power of that position.
- Then add up the results.
Example: 101011
- 1×25 = 32
- 0×24 = 0
- 1×23 = 8
- 0×22 = 0
- 1×21 = 2
- >1×20 = 1
So the sum is 32 + 0 + 8 + 0 + 2 + 1 = 43; thus, the decimal representation of (101011)2 is (43)10.
The traditional conversion from a decimal number to a binary number is straightforward but can be cumbersome. An even easier way to achieve this conversion is through BCD encoding.
Binary-Coded Decimal: Simplifying Decimal to Binary Conversion
Have you ever heard of Morse code? It's a very cool code that uses dots and dashes to represent letters and numbers. Just like Morse code translates human language into simple symbols that can be transmitted over telegraphs, Binary-Coded Decimal (BCD) acts as a bridge between our familiar decimal system and the binary system used by computers.
Why BCD?
The Binary-Coded Decimal (BCD) system represents each digit of a decimal number with its equivalent binary number. BCD makes the conversion from decimal to binary very easy and is thus favored in applications with many decimal arithmetic.
How BCD Works: The 8421 or 4-bit BCD Code
The most common BCD encoding is the 8421 or 4-bit BCD code, where each digit of a decimal number is represented by its 4-bit binary equivalent:
Decimal | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 |
Binary | 0000 | 0001 | 0010 | 0011 | 0100 | 0101 | 0110 | 0111 | 1000 | 1001 |
Table 1: Decimal digits and their binary equivalents
Here's the BCD encoding for the number 169:
- The decimal digit 1 corresponds to 0001 in BCD.
- The decimal digit 6 corresponds to 0110 in BCD.
- The decimal digit 9 corresponds to 1001 in BCD.
Putting them together, the BCD encoding for the number 169 would be:
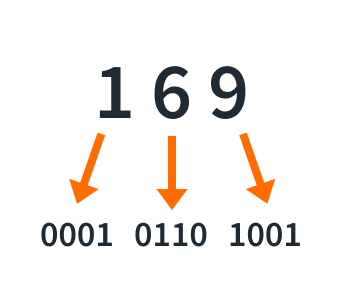
BCD Advantages and Uses
BCD's primary advantage is the fast and straightforward conversion between decimal and binary numbers, facilitating easy decimal arithmetic.
Also, BCD is commonly used in electronic devices that need to display numerical data, like digital clocks or calculators.
Why is this? Imagine a digital clock. If the time is 12:34, the clock must handle each digit (1, 2, 3, 4) separately to display it on the screen.
Since BCD treats each digit separately, it aligns well with how display hardware actually works.
BCD Limitations
Unlike a straight binary code representing the entire decimal number in binary, BCD converts each decimal digit individually. For example, representing the number 255 requires 12 bits in BCD, whereas only 8 bits are needed in straight binary.

This difference highlights BCD's main drawback: its inefficiency in storage. It occupies more space than a pure binary representation, leading to potential issues in memory-constrained systems.
Moreover, BCD calculations often require more complex circuits than pure binary, adding to the system's complexity.
Implementing Binary to BCD Conversion on Arduino
A code converter is a logic circuit or software function that transforms data from one format to another. Today we will implement the Binary to BCD conversion on the Arduino platform.
Decimal Number | Binary Number | Binary-Coded Decimal (BCD) |
0 | 0000 | 0000 |
1 | 0001 | 0001 |
2 | 0010 | 0010 |
3 | 0011 | 0011 |
4 | 0100 | 0100 |
5 | 0101 | 0101 |
6 | 0110 | 0110 |
7 | 0111 | 0111 |
8 | 1000 | 1000 |
9 | 1001 | 1001 |
10 | 1010 | 0001 0000 |
11 | 1011 | 0001 0001 |
12 | 1100 | 0001 0010 |
13 | 1101 | 0001 0011 |
14 | 1110 | 0001 0100 |
15 | 1111 | 0001 0101 |
Table 2: Decimal with their Binary and BCD equivalents
Decimal Binary BCD Conversion Table. Image Courtesy: digital detective
Why even bother learning this? Well, sometimes, you might need to convert binary numbers into a format that's easier to process or display. BCD format is suitable for displaying numbers on seven-segment displays or other digital readouts standard in Arduino projects.
The Arduino code below works as follows:
Step 1: Ask the user to enter an 8-bit binary number.
Step 2: Validate the number by checking if it is the correct length, 8 bit, and if every element is indeed binary.
Step 3: The conversion starts by first converting the input binary number to its decimal Equivalent
Step 4: Convert the decimal value to its BCD equivalent.
String userBinaryInput = ""; // Variable to store user's binary input
unsigned int userBinaryInputLength = 0; // Variable to store length of user's binary input
int bcdEquivalent = 0; // Variable to store BCD equivalent of binary input
int decimalEquivalent = 0; // Variable to store decimal equivalent of binary input
bool isBinaryInputValid = true; // Flag to validate user's binary input
// Variables to hold binary character representations
char binaryCharZero = char('0');
char binaryCharOne = char('1');
// BCD output will be stored in this array
byte bcdOutputArray[3];
int bcdArraySize;
// BCD lookup table for digits 0-9
const byte BCD_LOOKUP_TABLE[10] = {
0b0000, // 0
0b0001, // 1
0b0010, // 2
0b0011, // 3
0b0100, // 4
0b0101, // 5
0b0110, // 6
0b0111, // 7
0b1000, // 8
0b1001 // 9
};
// Function to convert decimal value to BCD representation
void convertDecimalToBCD(int decimalValue, byte *bcdOutputArray, int &bcdArraySize) {
// Ensure the buffer is clean
for (int i = 0; i < 3; i++) {
bcdOutputArray[i] = 0;
}
int index = 0;
while (decimalValue > 0) {
byte digit = decimalValue % 10; // Get the rightmost decimal digit
// If the index is even, store the BCD value in the lower half of the byte
// If the index is odd, store the BCD value in the upper half of the byte
bcdOutputArray[index / 2] |= (index % 2 == 0) ? BCD_LOOKUP_TABLE[digit] : (BCD_LOOKUP_TABLE[digit] << 4);
decimalValue /= 10;
index++;
}
// Ceiling division to find the size of BCD array
bcdArraySize = (index + 1) / 2;
}
// Function to print binary values
void printBinary(byte value) {
for(int i = 7; i >= 0; --i) {
Serial.print((value & (1 << i)) ? '1' : '0');
}
}
// Function to convert binary value to decimal
void convertBinaryToDecimal(String binaryInput){
int binaryValueProduct = 0 ;
for (int i = 7 ; i >= 0; i--){
int actualIndex = 7 - i;
int binaryValue = pow(2,actualIndex);
// If index is greater than 1, increment the value
if (actualIndex > 1){
binaryValue++;
}
// If the binary digit is 0, add nothing to the decimal equivalent
// If the binary digit is 1, add the binary value to the decimal equivalent
binaryValueProduct = binaryValue * (binaryInput[i] == binaryCharZero ? 0 : 1);
decimalEquivalent += binaryValueProduct;
}
// Display the decimal equivalent of the binary input
Serial.print("The decimal equivalent of ");
Serial.print(binaryInput);
Serial.print(" = ");
Serial.println(decimalEquivalent);
// Convert the decimal equivalent to BCD
convertDecimalToBCD(decimalEquivalent, bcdOutputArray, bcdArraySize);
// Reset decimal equivalent for the next conversion
decimalEquivalent = 0;
}
void setup() {
// Open Serial communications port
Serial.begin(9600);
// Display an introduction message
Serial.println("Binary to BCD Conversion");
Serial.println();
}
void loop() {
Serial.println ("Enter 8-bit binary number (e.g., 10011011): ");
while (Serial.available() == 0){
}
// Read input from user and display it
userBinaryInput = Serial.readString();
Serial.println(userBinaryInput);
// Check the length of the string to see if it is 8-bit long or not
userBinaryInputLength = userBinaryInput.length();
if (userBinaryInputLength != 8 ){
isBinaryInputValid = false;
Serial.println("Input binary number is not an 8-bit long binary number.");
Serial.print("Rather, it is: ");
Serial.print(userBinaryInputLength);
Serial.println(" long");
return;
}
// Check to see if all the elements of the input binary number are binary (0/1)
for (int i = 0; i < userBinaryInputLength; i++){
if (userBinaryInput[i] != binaryCharZero && userBinaryInput[i] != binaryCharOne){
Serial.println("Invalid Binary Number");
isBinaryInputValid = false;
break;
}
}
// If the binary input is valid, convert it to decimal
if (isBinaryInputValid){
Serial.println("I received a VALID BINARY INPUT");
convertBinaryToDecimal(userBinaryInput);
}
// Print the BCD output
for (int i = bcdArraySize - 1; i >= 0; i--) {
printBinary(bcdOutputArray[i]);
Serial.println();
}
}
In addition to using Arduino, you can experiment with a 74LS147 decimal-to-BCD IC to further explorenumber conversions.
The output snippet is here:
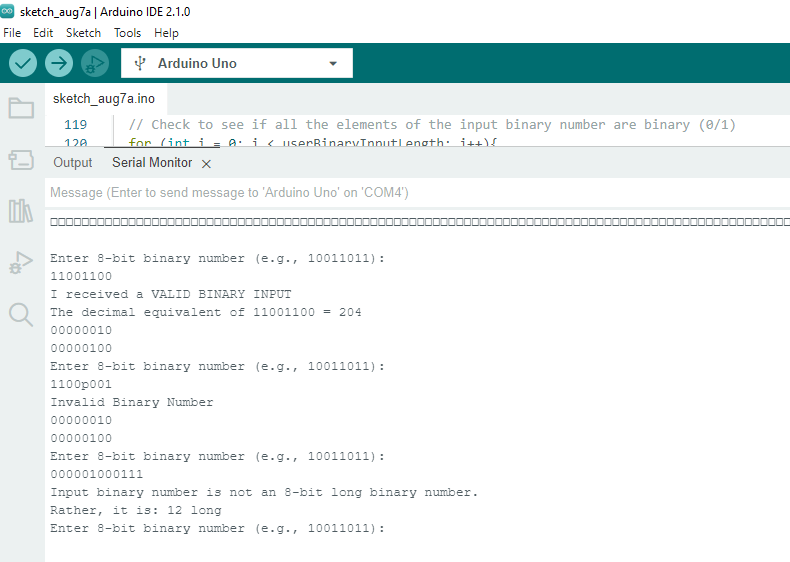
Conclusion
The binary number system is the backbone of digital circuits. It is simple and efficient. BCD, on the other hand, is a bit like a translation tool. It makes the conversion from decimal to binary very easy.
And, although BCD is less storage-efficient than pure binary, this trade-off allows more straightforward digital display calculations. Despite their differences, both systems have unique strengths that make them indispensable in their respective contexts.